二次订正版.
In order to run java, we need to download JDK(java development kit).
HelloWorld
Every java program need to have a class, all java code must inside a class.
1 | public class HelloWorld{ |
void是不return任何东西的key value.
Then save it to “HelloWorld.java”.
Java conventions require the file name to be the same as the class name.
Java跟C一样,得先compile再run.
在command line里.
1 | javac HelloWorld.java |
生成了HelloWorld.class file.
然后再同个directory里run.
1 | java HelloWorld |
在节约change->compile->change->compile之间,可以下载IDE来make life easier.
下载IntelliJ IDE.
感觉跟Xcode有点像(这个人并没用懂Xcode虽然).
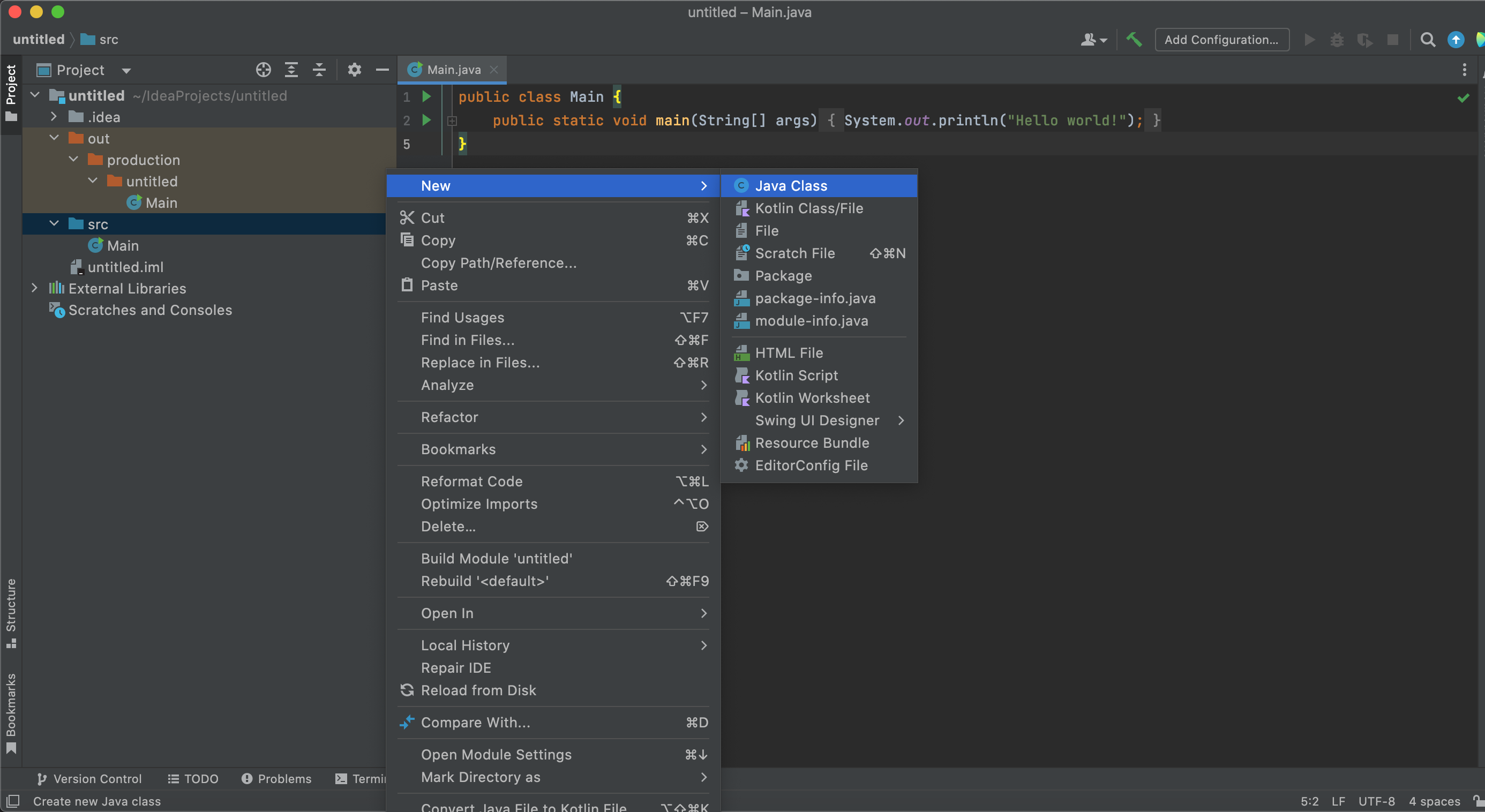
页面.
src存放java source code.
在src中新建java code(class).out folder是我build project的时候自动生成的.
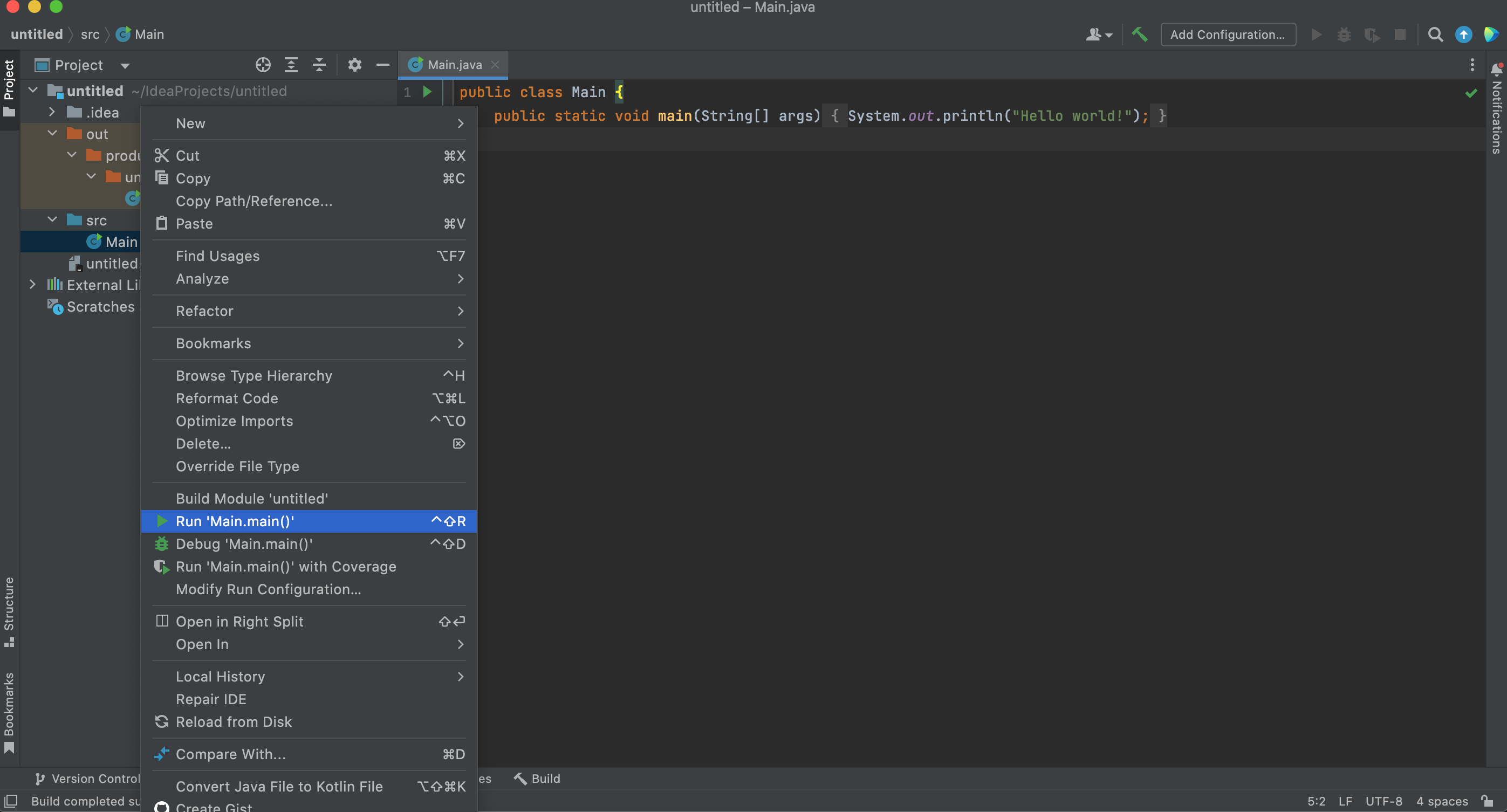
要跑起来只要run就行了.右上角的三角形也行.
Function
跟python不一样(?),java必须拥有main function.
1 | public class Main { |
跟c一样,pass data to function as argument的时候要写data type.
如果要return,需要declare return type.(void为不return)
1 | static int add(int first, int second){ |
在java中的argument可以是不定复数(varargs):
这时候pass进的是一个名为values的int array.
1 | static int add(int... values){ |
一个小tip:当一个function是return void的时候,可以考虑改成return boolean,给一个这个method是否worked.
Data type
跟c一样,得注明data type when initialization.
1 | public class Main{ |
如果数据只被initial没有reference(使用),在intellij中是灰色的.
Java中大小写matters.
String is built out of characters.
以及string的char index是从0开始的.
charAt是string operation,所以是后缀function.
Primitive types hold literal values(不像python全是ref), manipulated using Java operators (not methods), 比如+和-等等.
Primitive没有functionality.
如果primitive需要functionality, wrapper class.
Wrapper class is a designated object that contains a single primitive inside of it. All primitive have a wrapper.
Primitive Data Type | Wrapper Class |
---|---|
byte | Byte |
short | Short |
int | Integer |
long | Long |
float | Float |
double | Double |
boolean | Boolean |
char | Character |
在这些wrapper class里,有自带converter method,parser method.
命名提议:
Variable in dromedary case: somethingLikeThis
Constants: SOMETHING_LIKE_THIS
Data type chart:
Data Type | Size | Default Value | Description |
---|---|---|---|
byte | 1 byte | 0 | Stores whole numbers from -128 to 127 |
short | 2 bytes | 0 | Stores whole numbers from -32,768 to 32,767 |
int | 4 bytes | 0 | Stores whole numbers from -2,147,483,648 to 2,147,483,647 |
long | 8 bytes | 0L | Stores whole numbers from -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807 |
float | 4 bytes | 0.0F | Stores fractional numbers. Sufficient for storing 6 to 7 decimal digits |
double | 8 bytes | 0.0 | Stores fractional numbers. Sufficient for storing 15 decimal digits |
boolean | 1 bit | false | Stores true or false values |
char | 2 bytes | ‘\u0000’ | Stores a single character/letter or ASCII values |
Numeric Literals
Numeric type can added _ (underscore) to improve readability.
Can’t be used as first or last char of numeric literal, or either side of the decimal point.
1 | long underscordeLiteral = 2_000_000_000_000; |
Primitive Casting
If the data type on the right is smaller than the datatype ono the left, the conversion is implicit:
因为没有那么多type存,跟c一样.
Other way round there will be a explicitly cast.
1 | int intAge = 35; |
为了避免loss of data,我们可以:
1 | int intAge = 1; |
Input output
1 | import java.util.Scanner; |
String
Strings are object types that store text, is an array of characters.
Two ways of create:
1 | String name = "lan"; // declare as pure string |
Strings created using first method are stored in their own memory - the String pool, will be re-used wherever possible. String pool is a type of design pattern, known as object pool.
Pool : a section or memory where allows you to use existing values. 所以当有duplicate information的时候,比如有两个string variables contains the same word, the string pool will only have 1 instance of that word, and the two variables will point at the same object in memory.
Strings are immutable, when concatenation, a new string is always created.
Mutable refer to whether or not something can be changed.
Command function.
1 | String substring(int start) |
1 | String toUpperCase() |
1 | Char [] toCharArray() |
1 | String [] split(String regex) |
1 | String firstString = "Hello"; |
This way will point the same place in memory.
1 | String firstString = new String("Hello"); |
This will be two separate objects in memory.
于是发现在这里double equal compare的竟然是address instead of the value.可以compare primitive.
所以string comparison (或者objects)应该使用equal的method.
因为running java application的时候,memory会分为两块,一块是stack,一块是heap.
If here is a primitive variable, the stack will contain the literal value.
If the variable is an object, the stack will contain a pointer, a reference to the objects real location in the heap, because the stack is not big enough to actually store a full object.
Double equals(==) compares the value in the stack (就可能是pointer了). 两个不同的object 的地址是不同的所以false.
Array
跟c一样,array里面的variable需要same type,以及array size是immutable的.
Array基本没有自带function.
index从0开始.
1 | int[] numbers = new int[3]; |
From a pre-defined group of values:
1 | double[] prices = {1.25, 2.99, 1.99}; |
或者用生成的value.
1 | String sentence = “this is a sentence”; |
ArrayList is a collection that allows for elements to be added and removed, 像是dynamic array.比起array也有更多的function.
(Uses the Collections class instead of array class)
collection里的function比array的library还多,所以并不推荐使用array,function多会方便一些.
Inside the arrow heads is a class name that denotes what will be stored in the ArrayList.
Primitives cannot be between the arrowheads- only reference types.
1 | ArrayList<String> names = new ArrayList<>(); |
Common function:
get(index i) – gets the element at the index
set(index i) – sets the element at the index
remove(index i) – removes the element at the index
size() - returns the size of the array
toArray() – returns an array filled with the elements in the list.
Not a comprehensive list- check the Java ArrayList API for more
因为array自带的function太少,arrays和collections是addtional library:
Methods in the Arrays class include things such as sort(), fill() and binarySearch(), and toString(), while Collections methods include min(), sort(), max(), frequency().
1 | import java.util.Arrays; |
1 | import java.util.Collections; |
Condition
Comparison operator is same as c.不等于是**!=**.
1 | import java.util.Scanner; |
也用&&和||.
注意&的结果跟&&一样.但是&会把两个condition都跑一遍,而&&遇到第一个false第二个就不会跑了.
注意跟c一样,(跟python不一样),if condition里面的variable是有scope的.
在inputtedNum < 5里define的data在else里不能使用.
1 | import java.util.Scanner; |
1 | public void automatedPhoneService(int option) { |
switch跟c一样,记得要break.不然会自动跑下去.
Ternary.
1 | String result = (mark > 75) ? “pass” : “fail”; |
Loop
While loop:
1 | while (condition) { |
Do/While loop:
1 | do { |
The Do/While loop will execute the code block once, before checking if the condition is true, then it will repeat the loop as long as the condition is true.
For Loop:
1 | for (int i = 10; i <= 20; i++) { |
For/Each Loop:
1 | String[] allFruits = { "Apple", "Orange", "Banana", "Grape", "Cherry" }; |
An enhanced For Loop that will iterate through all the elements of a collection or array.
Cannot modify collection while iterating it.因为collection是可以remove&add的.
Break & Continue:
1 | //this loop will stop when the value of i is equal to 5: |
Continue stops the current iteration of a loop, and then continues with the subsequent iterations.
1 | //this loop will print all the numbers from 1 to 10 except the number 5 |
Class
A blueprint used to create objects.
跟method中的scope一样,variable defined in class是local的,不是global的.
Java中的gobal variable并不是一开始普通存在(待续).
The ‘new’ keyword is used to create an object from a class:
1 | Trainee trainee1 = new Trainee(); |
这里trainee1是一个指向trainee object的pointer.
1 | Trainee trainee1 = new Trainee(); |
如果我们在assign一个variable,他们都会是trainee的pointer.
Array & List elements are references as well.
class里的function又名methods.
class里的properties又叫做attributes.
We add the keyword static if the function does not use the attributes of a class, but still relates to the overall theme or idea of the class.
在同一个directory下的Main.java和StudentProfile.java文件.
Main的文件里并没有链接代码,好厉害…!
1 | public class Main { |
注意,StudentProfile的class成为了一种data type.
Class sample:
1 | public class StudentProfile { |
Constructor
The default constructor(每个class都会有default constructor) takes no arguments unless we overwrite it.
1 | new Trainee(); //no argument, default |
To overwrite it:
1 | public Trainee(String name, String stream) { |
A custom constructor has the same name as the class.
It has no return type.
When a custom constructor is written, the default constructor is no longer available.
Final
是class里的类似constant的keyword,一旦被set了就不能在更改.
1 | private final int CONSTANT_ATTRIBUTE; |
设定的两种方法:可以作为class blueprint里就设定的东西,也可以用constructor设定.
1 | public class Circle { |
1 | public class Trainee { |
Dependency
An object of one class relies on an object of another class.
在此之下有四种dependency:
Dependency
One class uses an object of another class as a method argument or return type.
在UML中以虚线箭头表示.
比如:
| Trainee |
| :————- |
| |
| + takeExam(Exam exam): int |
depends on ->
Exam |
---|
- questions: ArrayList |
+ getAllQuestions(): ArrayList |
btw, -是private field, +是public field(详见复习UML).
Association
One class has an object of another class as an attribute.
在UML中以实线箭头表示.
Classroom |
---|
- trainer: Trainer |
depends on ->
Trainer |
---|
- name: String |
Dependency Injection:
Will use a setter or a constructor to inject a dependency instead of defining the variable in the owing class, will set the variable afterwards. This will make code more flexible.
Setter method:
1 | Trainer trainer = new Trainer(); |
Or the constructor:
1 | Trainer trainer = new Trainer(); |
Aggregation
One class has multiple objects of another class as attributes.
(Association的ex版本)
Classroom |
---|
- trainers: ArrayList |
depends on ->
Trainer |
---|
- name: String |
在UML中以实线空心菱形表示.注意,菱形在classroom(owner)这头.
Composition
An object of one class belongs to an object of another class and can’t exist without it.
(Association的条件版本)
Academy |
---|
- classrooms: ArrayList |
depends on ->
Classroom |
---|
- name: String |
在UML中以实线实心菱形表示.
要达成这样的dependency,会使用java的scope特性:
1 | public class Academy { |
Classroom only exist inside of the Academy.
这样的nested class并不推荐,但是make sense for abstraction.
Static
The static keyword is used to denote that an attribute or method belongs to the class itself, rather than an instance of a class.
Methods, attributes, and even classes can be given the static keyword.
我们并不需要一个object of that class to use that method. 我们call it by name from the class.
Methods:
1 | public static void methodName() {} |
Variable:
1 | private static int variableName; |
注意,static因为是脱离了class的,使用会break abstraction的定义,使用时要注意.
Static variables and methods are accessed directly from the class:
1 | ClassName.methodName(); |
在UML中,static是underline.
Can not access a non-static method/variable from a static method.
Common use: static factory method.
A static method whose job is to generate instance of that class.
这是constructor一样的工作.但把如何跑constructor(一些限制)自己管理.
Interface
俺感觉interface跟class很接近.
Interface only reference functionality, does not represent data.(只有method,没有data field.)
Interface不可以有variable field但是可以有constant.
parent class -> child class & interface -> class的区别在于,前者child只能有一个parent,而后者的class可以implement多数的interface.
在UML中interface会自带<
(Broken arrow with solid head indicates a ‘Realisation’)
- All methods are implicitly public(Abstract, default & static)
- All attributes are implicitly public, final and static
- Don’t have constructors
Example:
1 | public interface Chargeable { |
在class的情况下key word是extend,interface是implements:
1 | public class Tablet implements Chargeable { |
Implements two的情况:
1 | public class PortableLamp implements Chargeable, Lamp {} |
Debugging
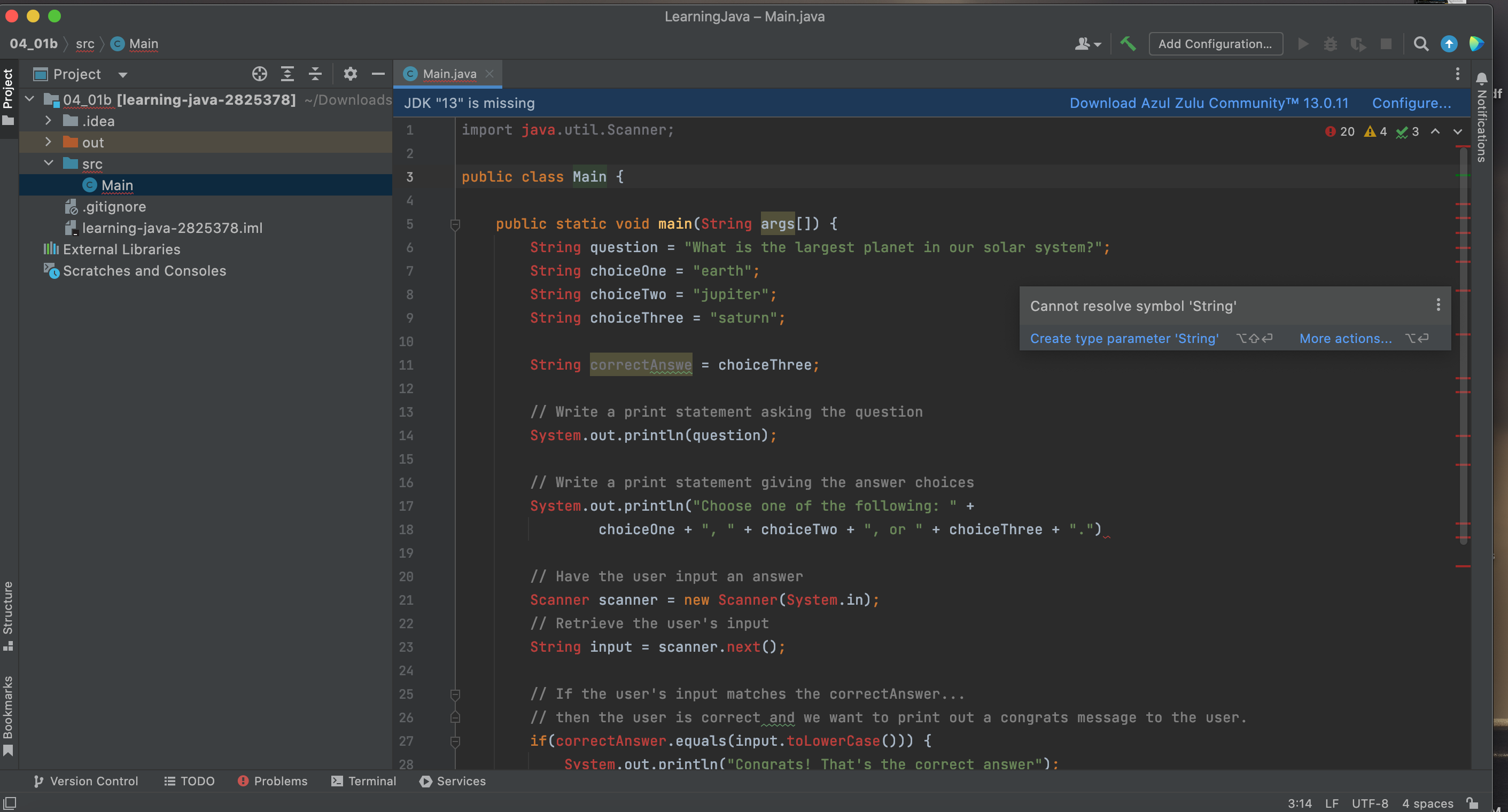
IntelliJ有一定程度的自动纠错,すごい.
Breakpoint is an intentional stopping point out into a program for debugging purpose.
With a breakpoint, we can temporarily halt a program in order to inspect its internal state.
点debug mode,然后创建breakdown point.
点有代码行数的左侧就行.再度run的时候会停下来告知variable当前value.
Casting
Upcasting
Accessing a child object using a reference of its parent class.
The parent reference points to the parent object within the child object.
Vehicle ref points to Car object 里的vehicle object.
Explicitly:
1 | Car car = new Car(); |
Implicitly:
1 | ArrayList<Vehicle> vehicles = new ArrayList<Vehicle>(); |
就是把这个car当作vehicle这个parent class使.
这个car中methods and attributes defined in the child class will not be accessible.
Code overriding methods defined in the parent class will be accessible.
Downcasting
反过来.
Downcast by specifying the correct type to downcast to:
1 | Car car = new Car(); |
The instanceof keyword is used to determine the correct type of an object.
Useful when downcasting objects stored in an ArrayList of the parent type.
1 | if (vehicle instanceof Car){ |
OOD
The 4 Pillars of OOD:
Encapsulation
Abstraction
Inheritance
Polymorphism
Encapsulation
Idea of bundling data and methods that work on that data within one unit-> class
We use Access modifiers to determine which objects can access attributes and methods of a class.
Default (no modifier) – any object of a class in the same package can access.
1 | int variableName; |
Public – any object can access.
1 | public void methodName(); |
Private – can only be accessed by methods within its own class.
1 | private int variableName; |
Protected – can be accessed by objects of classes in the same package and child classes.
1 | protected int variableName; |
Encapsulation的定义包括,让这个class负责它所负责的部分,所以应该优先于建造private method.优先度private -> default -> protected -> public.
但按照encapsulation的定义,把所有都private,还是有需要access某private field的需求,所以会做getters & setters function.
1 | private int week; |
(死去的c++记忆正在复苏…)
这一些是为了不让class裸奔(指使用public field).这样也方便设定get和set的限制.
Abstraction
We don’t need to know anything about the code within a method -> only knows the functionality.
可以理解abstraction是目的,而达成的方法是Encapsulation.
Inheritance
One class (the child) acquires the attributes and behaviours of another class (the parent)
(像之前谈过的dependency relationship)
AKA ‘Is A’ relationship:
Child -> Parent
Hawaii Pizza IS A Pizza.
The child is a more specific form of the parent.
The ‘extends’ keyword defines the parent of a class:
1 | public class Manager extends Employee {} |
- A class can only have one parent but multiple children
- A child class inherits all attributes and behaviours of its parent
- Constructors are not inherited
A child class must have a constructor which calls a constructor in its parent class:
1
2
3
4
5public Manager(String name, String jobTitle, int salary) {
super(name, jobTitle, salary);
}
super是用来call parent的constructor的.必须拥有super in child constructor还必须是first line of code.
If there is no custom constructor in the parent class then the default constructor in the child class will implicitly call the default constructor in the parent class. In this case no additional code needs to be written in the child class.
Protected
在parent里的protected 可以在child 里access:
(protect的用处,有点半private的意思)
Where a parent class attribute uses the ‘private’ access modifier, the child class will need to use getter and setter methods to access the attribute.(不能直接access)
If the parent class attribute uses the ‘protected’ access modifier, the child class can access it without needing to use the getter and setter methods.
在UML里protected是#.
Final
Final属性的class无法拥有child class(因为final的定义).
Abstract
Class中有一种是abstract,特定是并不会所有的functionality都define好,可能有placeholder,而在child中会把placeholder的部分补上.
因为parent是abstract的,我们无法create 一个concreate的parent.
1 | public abstract class Vehicle {} |
在vehicle里我们有一个abstract的method叫accelerate.
Abstract methods have no body:
1 | public abstract void accelerate(); |
在parent里只是这样decleare,没有implementation.
Child must be implemented in a concrete class which extends the abstract class:
1 | public class Car extends Vehicle { |
在UML中abstract的function是斜体(italic).
Polymorphism
Two types of polymorphism:
- Overriding
- Overloading
Overriding is related to inheritance, so if a method takes an object of type interface or abstract class, the exact child object passed into it will only become known when the code runs. Hence runtime polymorphism or late binding.
Overloading is related to the datatypes of the arguments passed into a method. This will be known when the code calling the method is written. Hence compile time polymorphism or early binding.
Overriding
Overriding很好理解,覆盖掉默认的method,比如child class要override parent class:
1 | public class Vehicle { |
在我们想保留parent method的情况下增加条件:
1 | public class Vehicle { |
这里的super是refer parent的setMaxSpeed.
这样先给car的speed设定条件,满足后再使用parent的method.
拒绝override可以使用final.
Overloading
Overloading的意思是multiple methods with the same name but with different arguments.
根据不同argument的data type来决定run哪个method.
1 | public class Car { |
Widening意味一些overload的type会被混淆,比如bytes shorts interger会混淆,应当避免.
Constructors can be overloaded:
1 | public class Car { |
Horizontal constructor chaining, arguments can be passed to a different constructor in the same class using this().
1 | public class Car { |
设置两个constructor,overload的使用原本的.
When used, this() must be the first statement in the constructor.