我为什么会写这个.
收到了一份亚马逊学生测试题,跟飞哥探讨了一下,又摸了下例题,选择放弃.
不过还是记载了.
翻例题答案时回想起自己也曾在python写过def main的function.
小葱拌豆腐行为,脑子清清白白.
上一次写纯python还是cs230或者cs231,那时还没有博客于是没有笔记记载,有些遗憾.
不知道这次图书馆更新结束后还会不会再续跟unity爱恨情仇的孽缘.
模拟题
今日嘉宾例题.
Find the missing number in the array
You are given an array of positive numbers from 1 to n, such that all numbers from 1 to n are present except one number x. You have to find x. The input array is not sorted.
1 | import random #random.randint() function |
这道题有意思的是:
The difference between these i.e. ‘expected_sum - sum_of_elements’, is the missing number in the array.
做了一个sum和difference.
Aside: Asset.
Determine if the sum of two integers is equal to the given value
Given an array of integers and a value, determine if there are any two integers in the array whose sum is equal to the given value. Return true if the sum exists and return false if it does not.
1 | def find_sum_of_two(A, val): # A is the array |
Aside: Set.
原来cs的set跟数学的set不一样啊!
set用于储存数据,好处可以guarantee search return O(1).
这里只做了一个array copy,然后比较差值是否在array中:从来看两数的sum是否在array里.
In this solution, you can use the following algorithm to find a pair that add up to the target (say val).
Scan the whole array once and store visited elements in a hash set.
During scan, for every element e in the array, we check if val - e is present in the hash set i.e. val - e is already visited.
If val - e is found in the hash set, it means there is a pair (e, val - e) in array whose sum is equal to the given val.
If we have exhausted all elements in the array and didn’t find any such pair, the function will return false.
Merge two sorted linked lists
好经典的题!
Given two sorted linked lists, merge them so that the resulting linked list is also sorted. Consider two sorted linked lists and the merged list below them as an example.
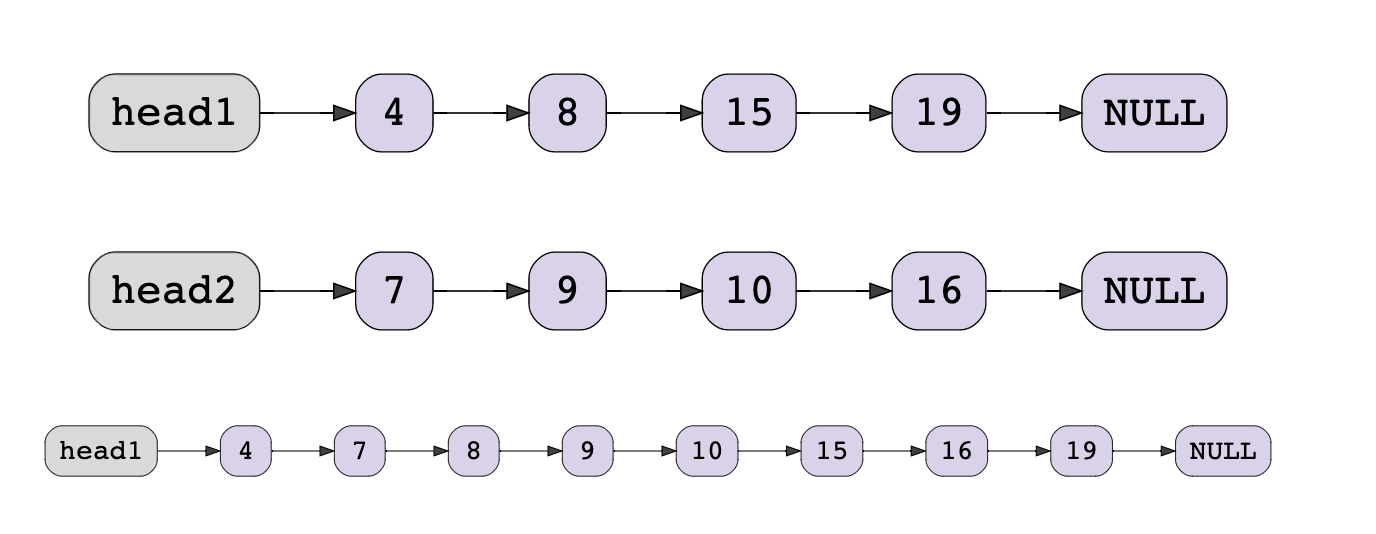
1 | def merge_sorted(head1, head2): |
Copy linked list with arbitrary pointer
You are given a linked list where the node has two pointers.
The first is the regular next pointer. The second pointer is called arbitrary_pointer and it can point to any node in the linked list.
Your job is to write code to make a deep copy of the given linked list. Here, deep copy means that any operations on the original list should not affect the copied list.
1 | def deep_copy_arbitrary_pointer(head): |
Level Order Traversal of Binary Tree
Given the root of a binary tree, display the node values at each level.
Node values for all levels should be displayed on separate lines.
Let’s take a look at the below binary tree.
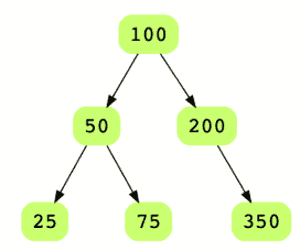
1 | # Using two queues |
Here, you are using two queues: current_queue and next_queue. You push the nodes in both queues alternately based on the current level number.
You’ll dequeue nodes from the current_queue, print the node’s data, and enqueue the node’s children to the next_queue. Once the current_queue becomes empty, you have processed all nodes for the current level_number. To indicate the new level, print a line break (’\n’), swap the two queues, and continue with the above-mentioned logic.
After printing the leaf nodes from the current_queue, swap current_queue and next_queue. Since the current_queue would be empty, you can terminate the loop.
然后放弃了思考
(测试的题目)
Reverse array queries
For a given array of integers, perform operations on the array. Return the resulting array after all operations have been applied in the order given.
Each operation contains two indices. Reverse the subarray between those zero-based indices, inclusive.
Example:
arr = [5, 3, 2, 1, 3]
operations = [[0, 1], [1, 3]]
In the first operations, reverse the subarray from arr[0] through arr[1]: arr’ = [3, 5, 2, 1, 3]
In the second operation, reverse the subarray from arr’[1] through arr’[3]: arr” = [3, 1, 2, 5, 3]
All operations have been performed, so return the array [3, 1, 2, 5, 3]
Function Description:
Complete the function performOperations in the editor below.
The function has the following parameter(s):
1 | int arr[n]: an array of integers |
Returns:
1 | int[n]: the final array after all operations have been performed |
Constraints:
1 | - 1 <= n, q <= 10^3 |
Input from stdin will be processed as follows and passed to the function.
The first line contains an integer, n, the size of arr.
Each of the following n lines contains a single integer, arr[i].
The next line contains an integer, q, the size of operations.
In the second line, there is a single integer 2, the number of columns in each operations[i].
Each of the following q lines contains two space-separated integers, operations[i].
Sample Case 0:
Sample Input:
1 | STDIN Function |
Sample Output:
1 | 2 |
Explanation:
The original array arr = [1, 2, 3].
Reverse arr[0] through arr[2]: arr’ = [3, 2, 1].
Reverse arr’[1] through arr[2]: arr” = [3, 1, 2].
Reverse arr”[0] through arr”[2]: arr’’’ = [2, 1, 3].
Sample Case 1:
Sample Input:
1 | STDIN Function |
Sample Output:
1 | 5 |
Explanation:
The original array arr = [5, 2, 5, 1].
Reverse arr[1] through arr[2]: arr’ = [5, 5, 2, 1].
Reverse arr’[1] through arr[1]: arr” = [5, 5, 2, 1].
Code workspace:
1 | #!/bin/python3 |
Validating Strings with RegEx
Given a list of strings made up of the characters ‘a’ and ‘b’, create a regular expression that will match strings that begin and end with the same letter.
Example:
‘a’, ‘aa’, and ‘bababbb’ match.
‘ab’ and ‘baba’ do not match.
Replacing the blank (i.e., “__”) with a regular expression that matches strings as described.
Locked code in the editor prints True for each correct match and False for each incorrect match.
Constraints:
- 1 <= query <= 10^3
- 1 <= |string| <= 10^3
- Each character string[i] belongs to {a, b}
Input Format for Custom Testing:
Input from stdin will be processed as follows and passed to the function:
The first line contains an integer query, the number of strings to be tested.
Each of the next query lines contains a string to validate.
Sample Case 0:
Sample Input:
1 | STDIN Function |
Sample Output:
1 | True |
Explanation:
The following query = 5 validations are performed:
- ‘a’ starts and ends with ‘a’, so it matches.
- ‘b’ starts and ends with ‘b’, so it matches.
- ‘ab’ starts with ‘a’ but ends with ‘b’, so it does not match.
- ‘ba’ starts with ‘b’ but ends with ‘a’, so it does not match.
- ‘aba’ starts and ends with ‘a’, so it matches.
Code workspace:
1 | #!/bin/python3 |
Precision and Recall
Given a 2-D matrix P of predicted values and actual values, write a function precision_recall to calculate precision and recall metrics.
Return the ordered pair (precision, recall).
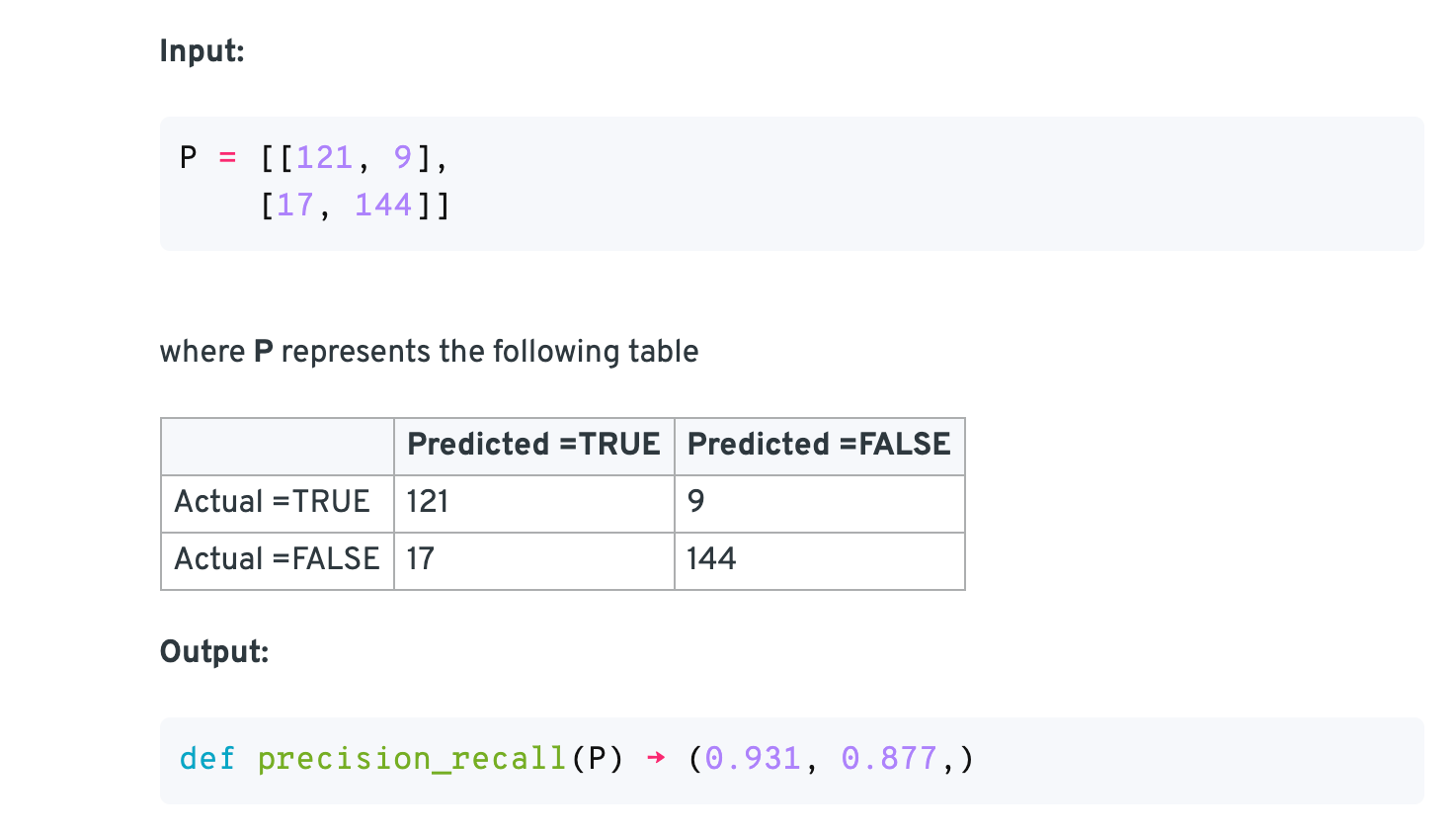
This problem tests your familiarity with a confusion matrix.
A confusion matrix represents is a simple 2x2 array where each entry is the number of True Positive, False Positive, True Negative, and False Negative data points from any given binary experiment. The positions of each data category are shown in the example.
Let’s recall the definitions of precision and recall.
The formula for calculating precision is as follows:
The precision value decreases as the number of False Positives increases. A lower FP value means our experiment was more precise.
The formula for calculating recall is:
The recall value decreases as the number of False Negatives increases. A False Negative indicates that we rejected a data point when we shouldn’t have and thus it should be recalled.
Once we are clear on the definitions of precision and recall, the problem is very easy to solve. We simply grab the relevant values from our input array P and plug them into the formulas which define precision and recall.
Our final code is as follows:
1 | def precision_recall(P): |
Alternates the cases of every alphabetic characters
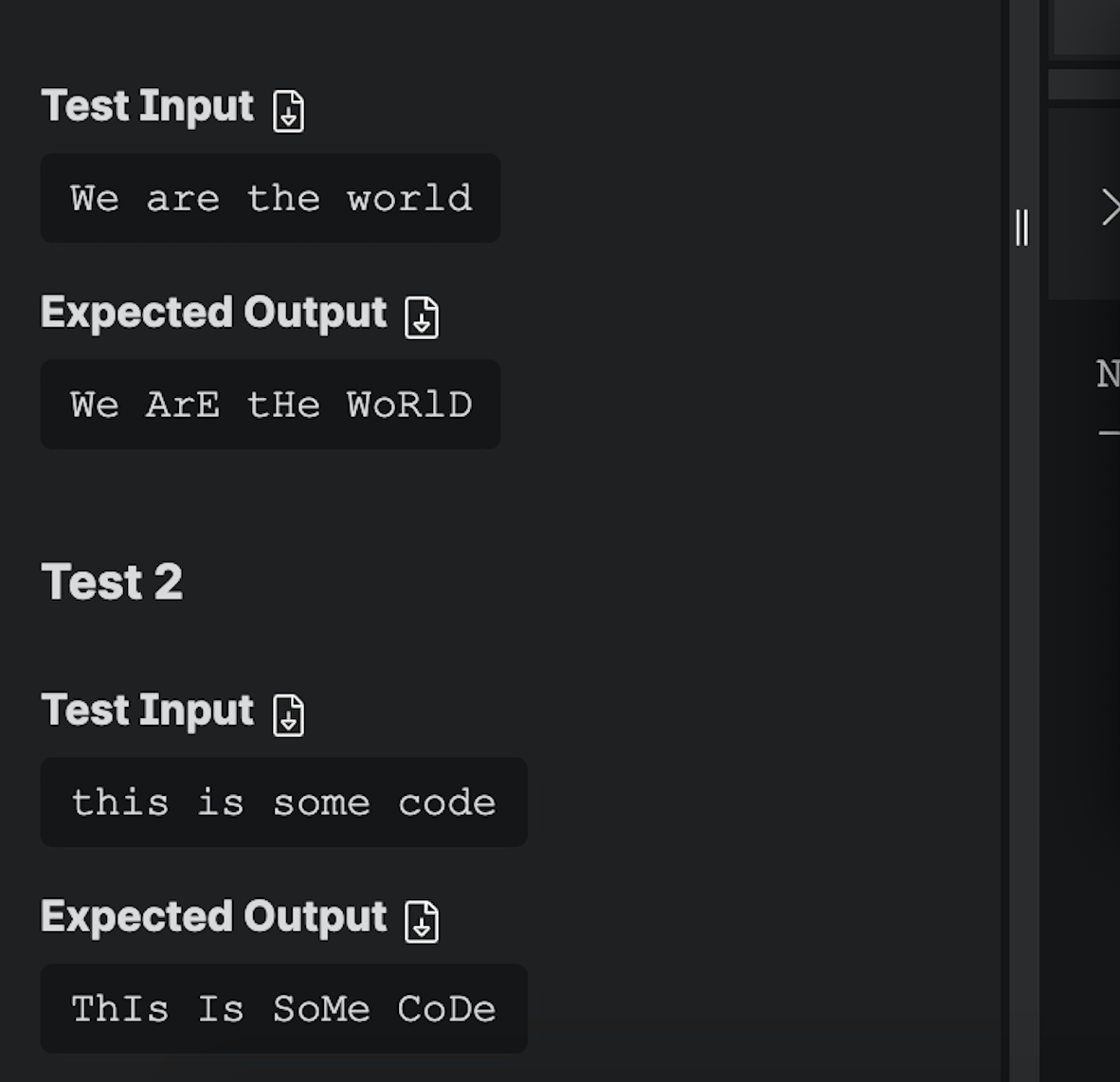
这道题,我使用了set,用loop读取每个char,如果是alphabet就变更.用一个bool来记录要不要upper.
Check duplicates
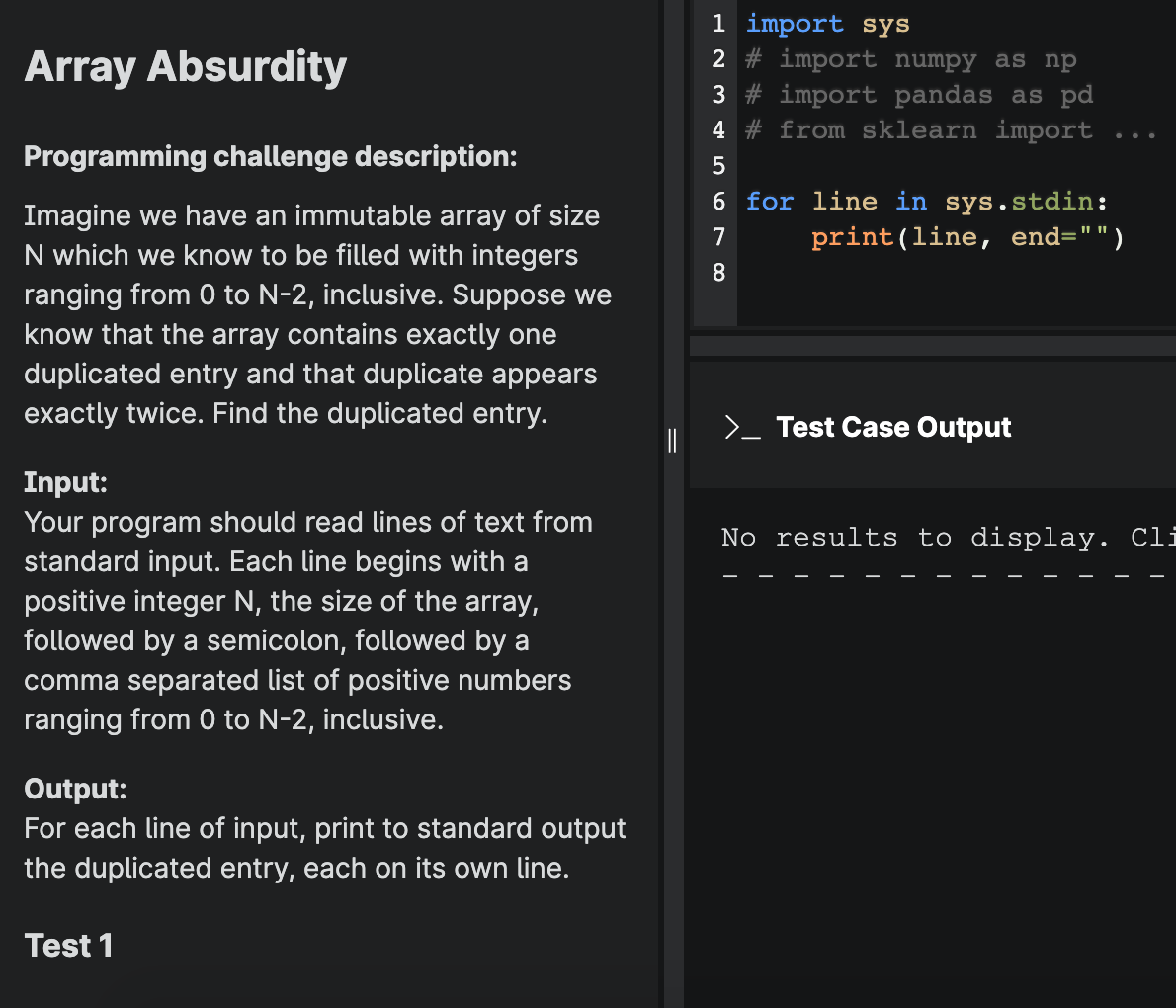
这道题比较经典,在stackoverflow上有找到.
Balanced smileys
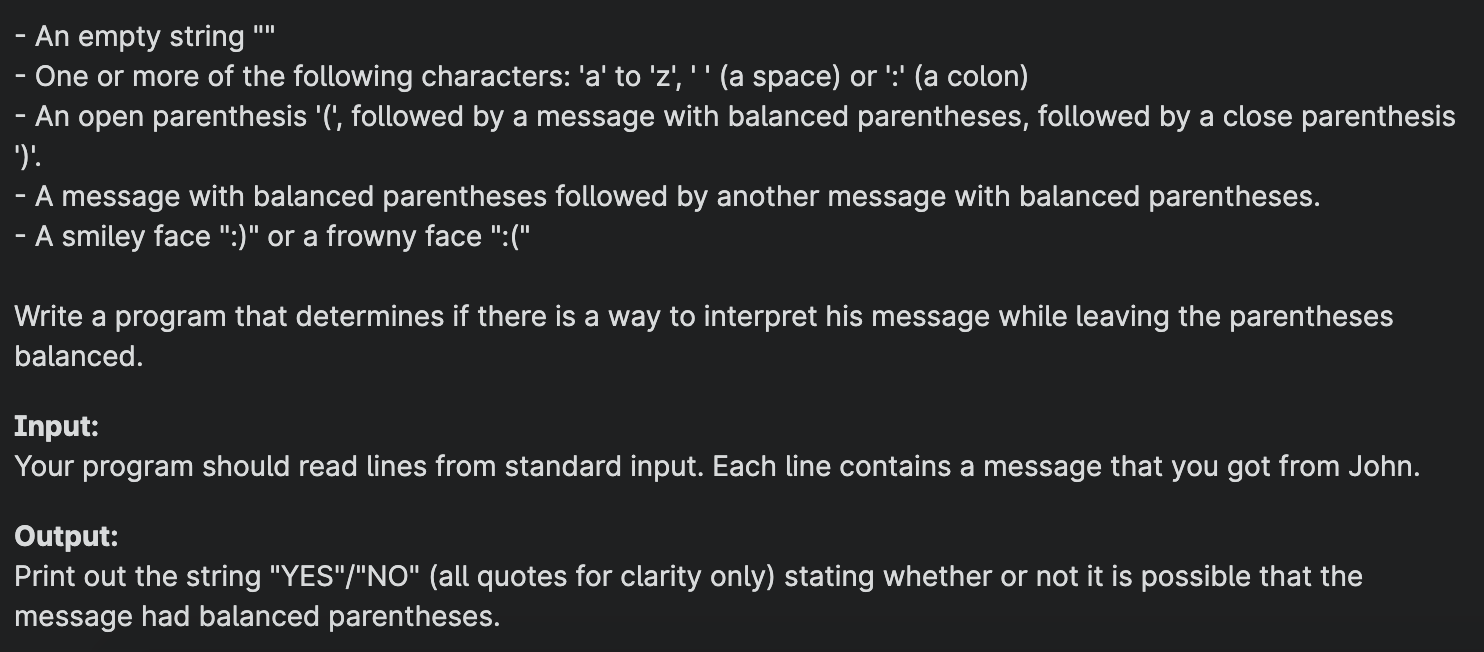
这道题有点烦.但是有出处.
笔记
Basic
在LinkedIn上听python最基础的课程.
因为当年是一周内把基础语法扫过,直接开始整题.
现在补一点基础笔记.
Shebang
1 | #!/usr/bin/env python3 |
演示的视频把这一行写在了python script的第一行,也就是让环境去跑python.我不记得当年是怎么写python了,现在用jupyter,就不用shebang code了.
Scope
Python是用indention来区分block的.但不同block内define的variable,不同block都可以用.
原句:block does not define the scope of variables in Python.
但是functions, objects, modules do define scope in Python.
1 | x = 42 |
- Main
1 | def main(): |
Class
Class里的function会把self给扔回去.
1 | class Duck: |
Type
Python uses a form of dynamic typing, sometimes called duck typing where the type of a value is determined by the value itself.
不像c语言,define一个variable会先写出variable的type.
python就直接写x = theValue.
然后跟着value的值,x成为不同的type.
关于precision python也有这个问题.
x = .1 + .1 + .1 - .3不等于零,会有一个小小小小的数.
为解决这个问题.
1 | from decimal import * |
可以使用decimal class.
Bitwise operators
1 | & : And |
Loop
1 | secret = 'swordfish' |
Function
All function return a value, if not specified, then return [None].
Call by value:
When you pass a variable to a function, the function operates on a copy of the variable, the value is passed, but not the object itself.
就比如说在c里面.Function(a).
function里reassign a to 5,不会改变a是3的事实.
但python不同.Python全部都是pointer,python会把reference(指向实际的数值)pass过去.
Integer(in python) is not mutable, it cannot change when you assign a new value to an integer, you’re actually assigning an entirely different object to the name. The original integer is not changed. The name simply refers to a new object.
然而integer又又是个特例.Integer不会给reassign.
所以比如数值是存在array里的,就会被改变了.
Passing a value to the function acts exactly the same way. A reference of object has passed and acts exactly like an assignment.
A generator is a special class of function that serves as an iterator. Instead of returning a single value, the generator returns a stream of values.
1 | #!/usr/bin/env python3 |
这里对yield的解释:yield is like return but for generator.
Yield
Omake之遇到一个data analyst相关的面试测试,要求写python.
以为是pandas/numpy相关,结果一打开:卧槽纯python.
不过比较简单,稍微查了下,用到了没用过的yieldfunction.
我理解为跟C中的std:in相似.