可惜上一次记载unity笔记的时候还没有使用博客.
纸质材料跟我的C++入门课一起静躺在书架上,不在脑内也不舍得丢弃.
来都来了,记点记点.
个人用,杂乱,请见谅.
Ctrl + K + C : Comment out the selected code.
Ctrl + K + U : Uncomment the selected code.
Ctrl + / : Toggle line comments.
Shift + Space -> 将鼠标所在的unity窗口放大.
Lighting
Light好像只能4个,再多会唐突消失在camera内.
Skybox
Skybox很好看,像是真实照片(背景)所做的lighting.还有自带的360度照片.
需要Create Skybox Material first, shader as Skybox/Panoramic, drag hdr file into it(the skybox).
(右手边的)Lighting -> Environment -> Skybox Material.
GameObject
Camera
Click on Main Camera -> GameObject -> Aligh with View,可以快捷调节camera的view.
Click on any window, shift space可以放大window.
Scrolling Repeating Background in Unity.
Sound
Camera default to have audio listener, but we can’t have 2 audio listener in scene, if we have two camera, remove one.
Background sound -> audio source -> 3D sound settings -> min distance set to 0, there is no minimum distance the user need (they always hear).
Post Process volume
很酷的一种visual滤镜.
首先要现在window(菜单),package manager,添加post processing.
Volume -> global volume + camera -> post processing turn on
Under global volume(object created) create new profile.
Then add override, vignette, intensity for testing. Bloom, tone mapping is fun too
effect -> particle system.
Collision
For trigger object(碰撞trigger的情况), 2 objects(collision) all needs rigid body into it.
Collision有两种.一种是“碰撞”,一种是"接触".
1 | private void OnCollisionEnter2D(Collision2D collision){ |
1 | private void OnTriggerEnter2D(Collider2D other){ |
这一种一般用于”路径点”,拾取道具.
需要在Collider 2D中turn on “Is trigger”.
Rigid body跟character control好像不兼容(需要查询).
Character control可以控制更多. -> 如果要使用on trigger,因为需要二者之一的rigid body所以对面需要拥有rigid body.
Canvas
要查看canvas的种类,在canvas -> render mode中查看.
如果是screen space - camera, 或者screen space - overlay -> 会让canvas的位置根据camera变动.
如果是world space -> 可以rename成world canvas,是一个canvas物体,跟其他物品一样.
要加入canvas中的物件.推荐使用这个小窗口.
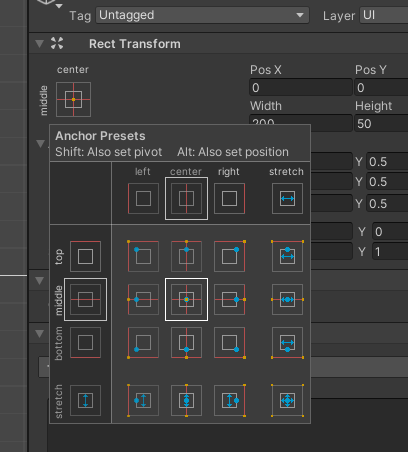
Left button selection + alt + shift, place object in one of default position.
Frame canvas -> canvas scaler-> UI Scale Mode, scale with screen size.
Sprite renderer renders a sprite in the sccene, UI image renders a sprite in UI.
Animator
如果需要给game object增加动画,需要添加animator component.
Animator需要animation controller(assets).
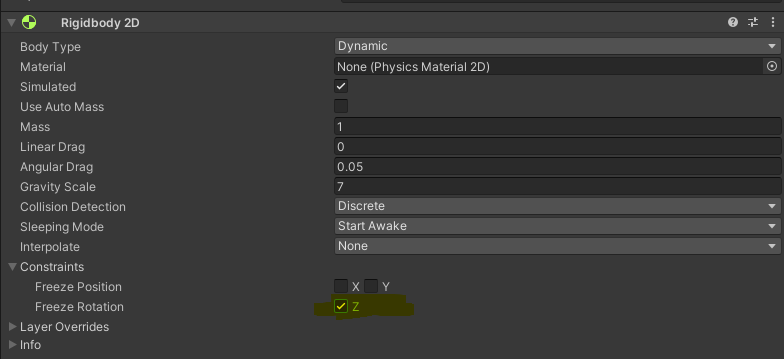
一个造门的小妙招.
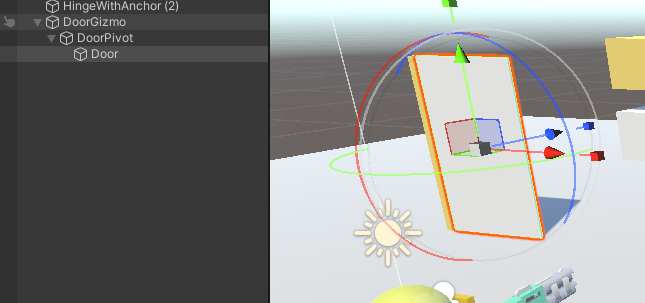
创造一个空的game object parent,然后再造一个door.
Gizmo的position正好在门要转动的轴上,所以只要用代码转动Gizmo就能转动门.
用两层parent是因为,一层用来转动,一层用来移动.
Joint
https://www.youtube.com/watch?v=MElbAwhMvTc&ab_channel=DitzelGames
https://www.youtube.com/@JamesMakesGamesYT/videos
https://www.youtube.com/watch?v=dEtuAPo5vAg&ab_channel=ChristopherFrancis
Input
1 | if (Input.GetKeyDown(KeyCode.Space)){ |
关于博主把GetKeyDown和GetKey混着用的这件事.
GetKeyDown如题只记录Down这个action是否实施过.
GetKey而是get key的这段时间一直都会return true.
Object Pooling
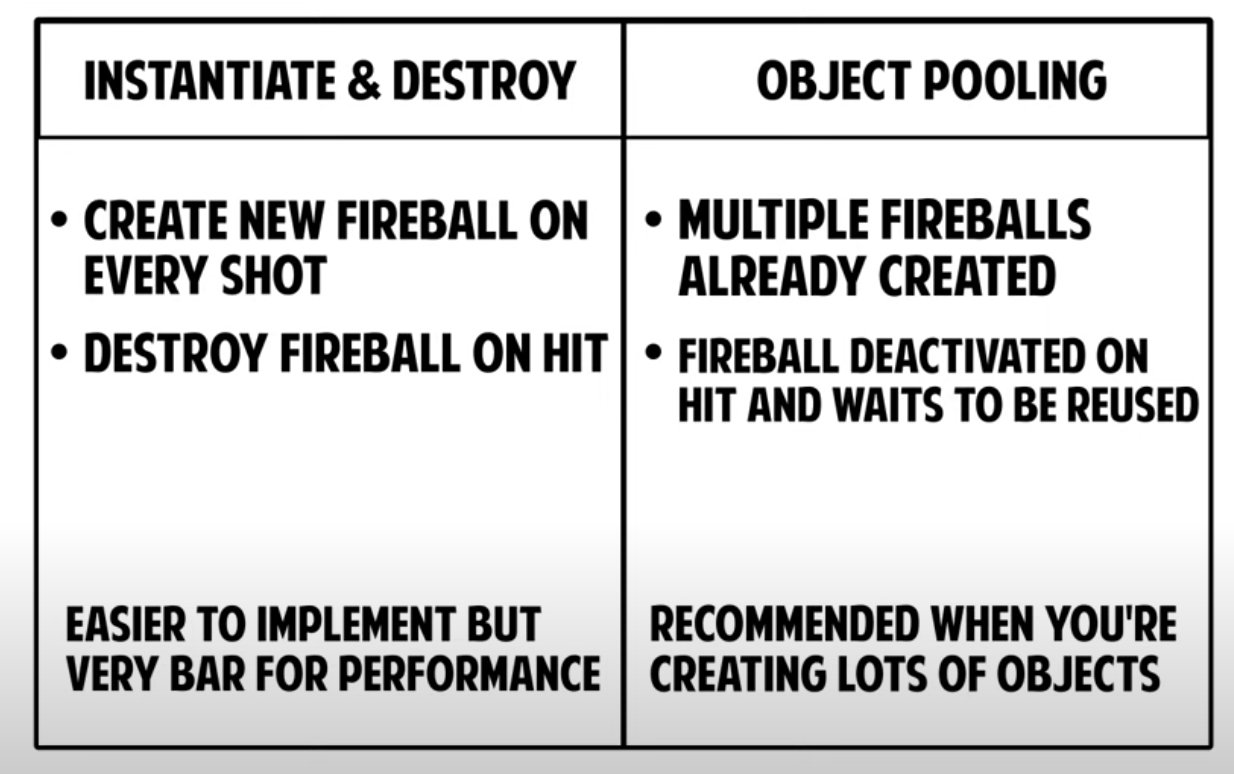
Sprite Multi
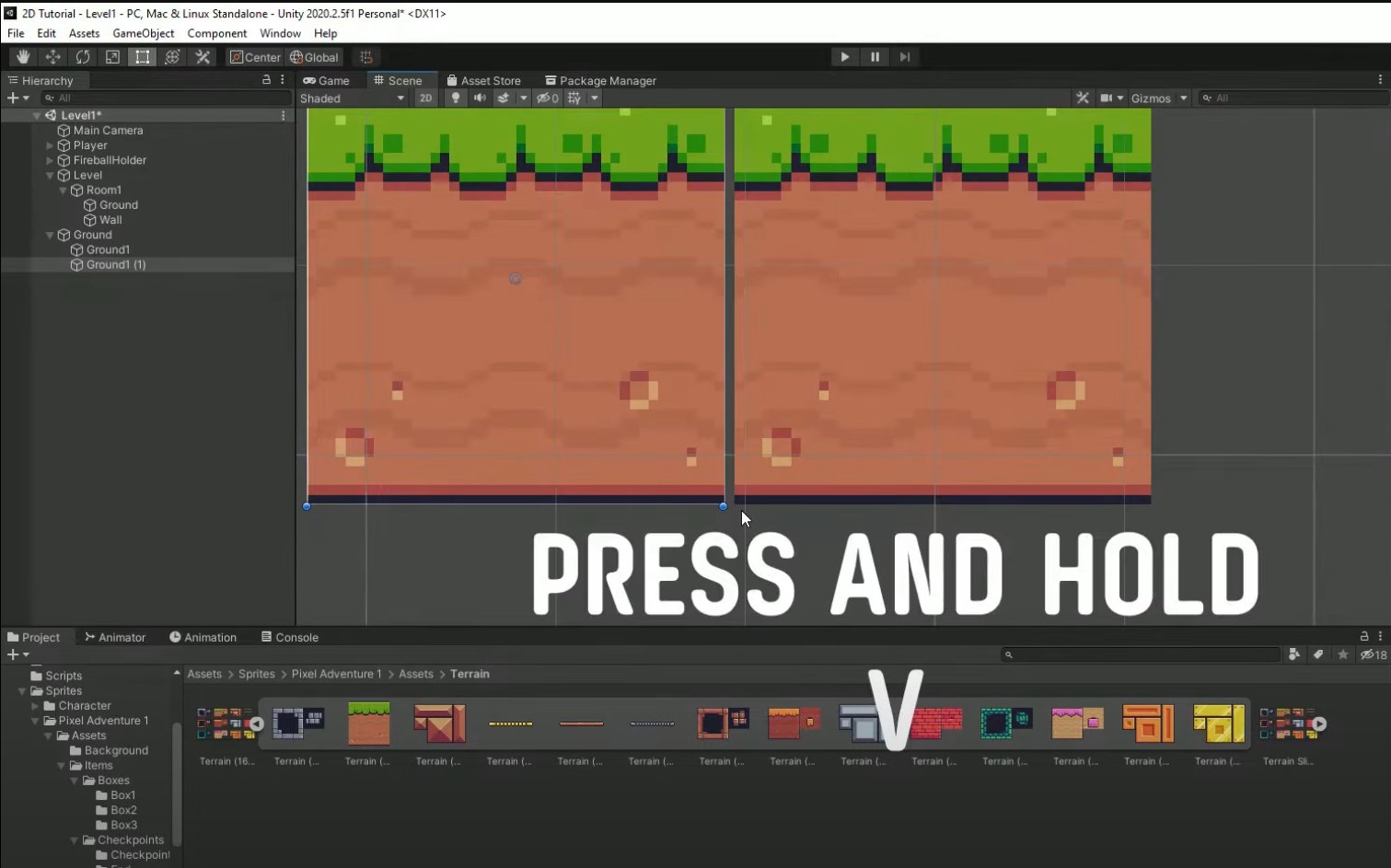
OOP Principles
Encapsulation: Bundling data and methods into a single unit (class) for organized code.
Inheritance: Allows a class to inherit properties and behaviors from another class, promoting code reuse.
Polymorphism: Objects can take multiple forms, enabling a single interface for different data types.
Abstraction: Simplifying complex systems by modeling classes based on essential properties.
只有abstract或者virtual才能override.
Encapsulation
1 | public class Health{ |
Inheritance
1 | public abstract class Car{ |
Polymorphism
1 | public class Animal{ |
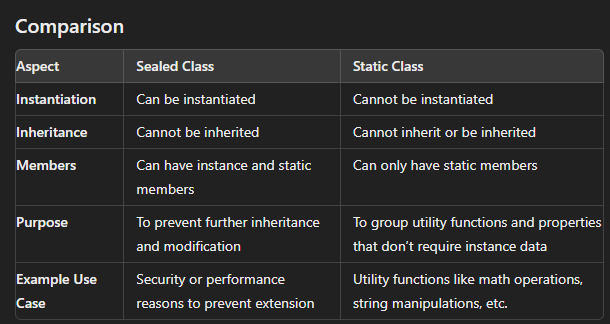
Abstraction
1 | public abstract class PlayableObject: MonoBehaviour, IDamageable |
Interfaces/what to use(使用方法类似于class,但只有declare没有definition) -> can use multi interfaces into one class.
Abstract class -> only declare no definition.
Virtual you can define.
1 | public class Enemy: PlayableObject{ |
Static的好处是static class下面的function到哪里都可以用.
一般需要getComponent读取object,但static就不用.
1 | public static class ConstantExample{ |
Custom Module
有几种比较便利的,常用class整理方法.
PlayableObject
IDamageable
GameManager
LevelLoader
SceneManager
ScoreManager
SoundManager
UIManager
比较方便的data写法.
- Array
- List
- Stack
- Dictionary
1 | public GameObject[] array = new GameObject[2]; |
List is dynamic but array is not. (and least performance).
Array holds same type of object. (string array only holds strings).
1 | public GameObject testPrefab; |
Stack: last in first out. (Use in backtracking, search history, undo behavior).
1 | public Stack<GameObject> stack = new Stack<GameObject>(); |
Queue: first in first out. (Use in order, schedule list, request).
1 | public Queue<GameObject> queue = new Queue<GameObject>(); |
Dictionary: Phonebook, user section (user id), inventory.
1 | using TMPro; |
Singleton
We can only have one singleton (therefore it called singleton).
Be careful for the variable in singleton (since they can only have one).
Eg.GameManager:
1 |
|
1 |
|
Delay
Unity timer IEnumertator.
//something wanna do before the wait
yield return new WaitForSeconds(0.5f);
//something wanna do after the wait
1 | StartCoroutine(EnemySpawner()); |
除了IEnumertator,还能用Invoke.
1 | Invoke("theFunctionName", 3);//3 seconds then the function |
IDamageable
以我的理解,用interface(contract)来限制所有增加的class都会有其interface内的内容.
像给div增加class一样可以一起使用.
1 | public interface IDamageable{ |
1 | private void OnTriggerEnter2D(Collider2D collision){ |
Solid Principle
Single responsibility
Open–closed
Liskov substitution
Interface segregation
Dependency inversion
Comment
1 | 连打三条杠(///). |
Using Interfaces in Unity Effectively | Unity Clean Code
An Automated Model Based Testing Approach for Platform Games
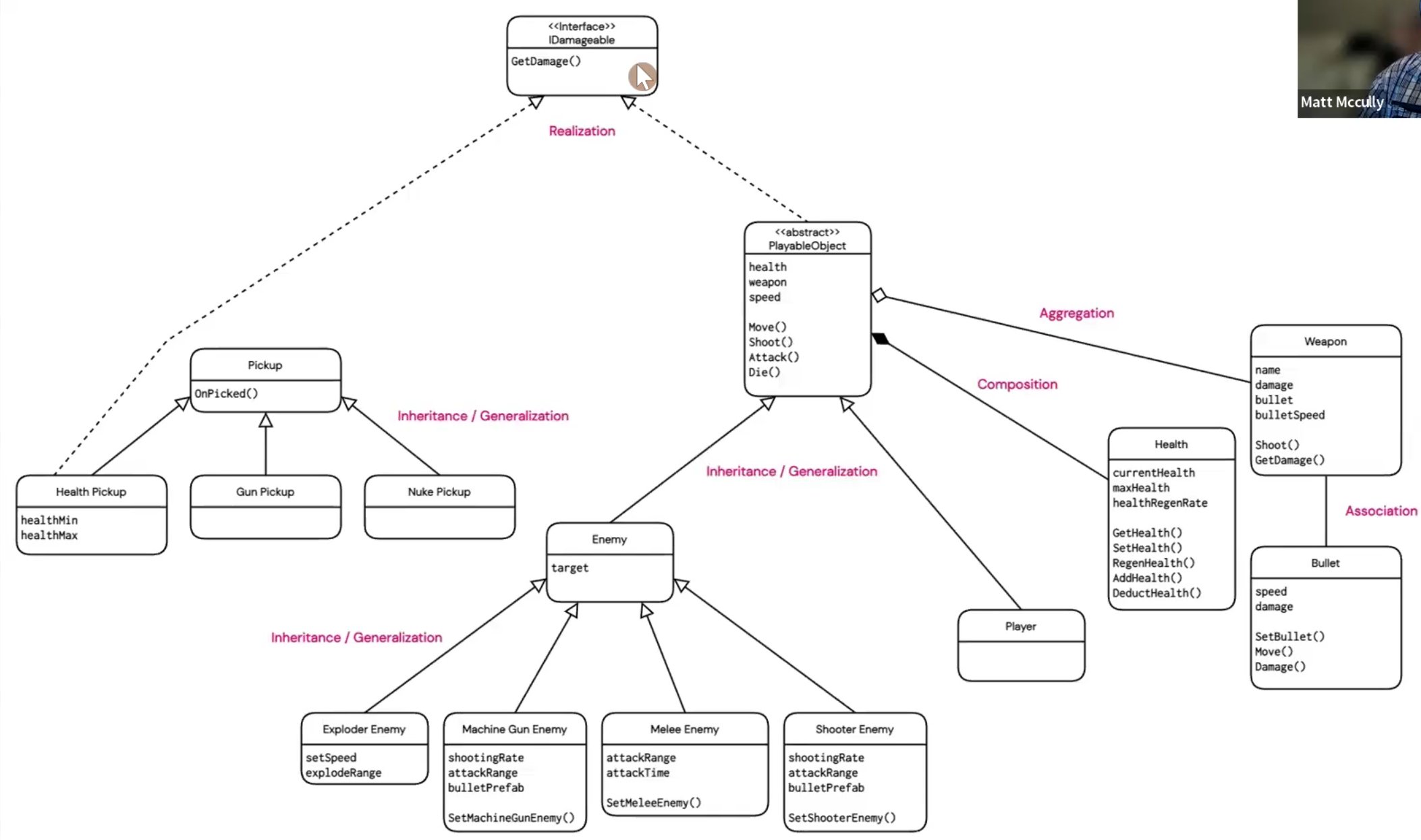
shader
Package Manager.
注意title选择Packages: Unity Registry.
Find Shader Graph.
Create -> Sharder Graph -> URP -> Lit Shader Graph/Unlit Shader Graph.
做好的shader要先attach to material.再material attach to the plane(or other stuff).
Builds
For building in different platforms.
Project setting -> player -> configuration -> scripting backend
mono is fine for testing, but for deliver version should choose IL2CPP.
For Api Compatibility Level -> .NET Standard 2.1 is fine.
don’t build project in repository, will blow up it, just share source code.
Add-on
ProBuilder
Strip All ProBuilder Scripts in Scene.
一款捏简单3D物体的plugin.
VRoid
How to Export VRoid Studio Models to Unity (3D/URP)
Game Programming Patterns
https://www.c-sharpcorner.com/UploadFile/damubetha/solid-principles-in-C-Sharp/